Presentation comments ...
--- ## Use modules for organization ```js ``` <details class="caption slides-small"> <summary>References</summary> - w3schools [modules](https://www.w3schools.com/nodejs/nodejs_modules.asp), [http module](https://www.w3schools.com/nodejs/nodejs_http.asp), [file system module](https://www.w3schools.com/nodejs/nodejs_filesystem.asp), [url module](https://www.w3schools.com/nodejs/nodejs_url.asp) </details> --- ## Testing **Overview**: How to use linters and write unit and integration tests ```js // 1. add and use custom module const fortune = require('./lib/fortune'); app.get('/about', (req, res) => { res.render('about', { fortune: fortune.getFortune() }); }); // 2. ``` #### Homework - [Brown](https://www.oreilly.com/library/view/web-development-with/9781492053507/): - Ch5 Quality Assurance (41–58) - Exercise: Add testing to Meadowlark Website --- ## Requests and Templating **Overview**: How to ... Express request, response, Handlebars, MVC ```js const tours = [ { id: 0, name: 'Hood River', price: 99.99 }, { id: 1, name: 'Oregon Coast', price: 149.95 }, ]; app.get ('/api/tours', (req, res) => res.json(tours)); ``` - Lecture: [Node, Express, Handlebars, Heroku - Part 1 - Set up a node express project template ](https://docs.google.com/presentation/d/17bIeMMJnZQy-tb3GLhMC3JuTfcgMEOqnK4WsCt52CLM/edit#slide=id.gafb807d421_0_39) - Demo: [omundy/sample-node-express-cat-api](https://github.com/omundy/sample-node-express-cat-api) and ~~[live demo](https://sample-node-express-template.herokuapp.com/)~~ #### Homework - [Brown](https://www.oreilly.com/library/view/web-development-with/9781492053507/) - Ch6 Request and Response (59-72) - Ch7 Templating with Handlebars (73-88) --- ## Forms and Sessions **Overview**: How to ... Express web forms, sessions ```js app.get ( '/newsletter', handlers.newsletter ); app.post ( '/api/newsletter-signup', handlers.api.newsletterSignup ); ``` - Lecture: [Node, Express, Handlebars, Heroku - Part 2 - Use API data, add a frontend](https://docs.google.com/presentation/d/17bIeMMJnZQy-tb3GLhMC3JuTfcgMEOqnK4WsCt52CLM/edit#slide=id.gbcac1199e9_0_15) - Demo: [omundy/sample-node-express-username-generator](https://github.com/omundy/sample-node-express-username-generator) and [live demo](https://sample-node-express-username.herokuapp.com/) #### Homework - [Brown](https://www.oreilly.com/library/view/web-development-with/9781492053507/) Ch8 Form Handling (89-100) & Ch9 Cookies and Sessions (103-112) ---  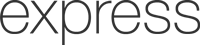 # Node Express Part.2 Using Node, Express for server-side, desktop, and mobile application development --- ## Express and databases **Overview**: How to ... ```js const mongoose = require ( 'mongoose' ); const { connectionString } = credentials.mongo; mongoose.connect ( connectionString ); ``` #### Homework - [Brown](https://www.oreilly.com/library/view/web-development-with/9781492053507/) Ch13 Persistence (147-172) #### Review - [MVC](https://en.wikipedia.org/wiki/Model%E2%80%93view%E2%80%93controller), [Revealing Module](https://gist.github.com/zcaceres/bb0eec99c02dda6aac0e041d0d4d7bf2#file-revealing-module-pattern-md), and other [Javascript Design Patterns](https://addyosmani.com/resources/essentialjsdesignpatterns/book/) --- ## Advanced routing ```js app.get ( '/user(name)?', ( req, res ) => res.render ( 'user' )); app.get ( '/staff/:name', ( req, res ) => { const info = staff [ req.params.name ]; if ( !info ) return next (); // will eventually fall through to 404 res.render ( 'staff', info ); }); ``` <details class="caption slides-small"> <summary>References</summary> - [Brown](https://www.oreilly.com/library/view/web-development-with/9781492053507/) Ch14 Routing (173-184) & Ch15 REST APIs and JSON (185-192) </details> --- ## Express SPAs **Overview**: How to ... ```js import React from 'react'; // ... ``` <details class="caption slides-small"> <summary>References</summary> - [Brown](https://www.oreilly.com/library/view/web-development-with/9781492053507/) Ch16 Single-Page Applications (193-212) </details> --- ## Production <details class="caption slides-small"> <summary>References</summary> - [Brown](https://www.oreilly.com/library/view/web-development-with/9781492053507/) Ch11 Sending Email (121-131) & Ch12 Production Concerns (133-145) </details> --- ## Express security, APIs **Overview**: How to ... ```js const passport = require ( 'passport' ); const db = require ( '../db' ); // ... ``` #### Homework - [Brown](https://www.oreilly.com/library/view/web-development-with/9781492053507/) - Ch18 Security (223-248) - Ch19 Third Party APIs (249-263) --- ## Express debugging, production **Overview**: How to ... ```bash node inspect index.js ``` #### Homework - [Brown](https://www.oreilly.com/library/view/web-development-with/9781492053507/) - Ch20 Debugging (265-275) - Ch21 Going Live (277-288) - https://heroku.com/ - Ch22 Maintenance (291-300)